How to use the DateTimeSpinner component (Starling version)
The DateTimeSpinner
component allows the selection of date and time values using a set of SpinnerList
components. It support multiple editing modes to allow users to select only the date, only the time, or both.
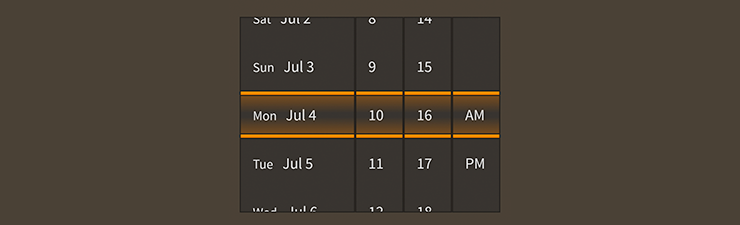
DateTimeSpinner
component skinned with MetalWorksMobileTheme
The Basics
First, let's create a DateTimeSpinner
control, set up its editing mode and its range of values, and add it to the display list.
var spinner:DateTimeSpinner = new DateTimeSpinner();
spinner.editingMode = DateTimeMode.DATE;
spinner.minimum = new Date(1970, 0, 1);
spinner.maximum = new Date(2050, 11, 31);
spinner.value = new Date(2015, 10, 31);
this.addChild( spinner );
The value
property indicates the currently selected date and time, while the minimum
and maximum
properties establish a range of possible values. You may omit the minimum
and maximum
properties, and reasonable defaults will be chosen automatically.
The editingMode
property determines how the date and time are displayed.
DateTimeMode.DATE
displays only the date, without the time. The month and day are displayed in order based on the current locale.DateTimeMode.TIME
displays only the time, without the date. The time is displayed in either 12-hour or 24-hour format based on the current locale.DateTimeMode.DATE_AND_TIME
displays both the date and the time. As with the previous mode, the current locale determines formatting.
Add a listener to the Event.CHANGE
event to know when the value
property changes:
spinner.addEventListener( Event.CHANGE, spinner_changeHandler );
The listener might look something like this:
function spinner_changeHandler( event:Event ):void
{
var spinner:DateTimeSpinner = DateTimeSpinner( event.currentTarget );
trace( "spinner.value changed:", spinner.value );
}
Skinning a DateTimeSpinner
The skins for a DateTimeSpinner
control are divided into multiple SpinnerList
components. For full details about which properties are available, see the DateTimeSpinner
API reference. We'll look at a few of the most common properties below.
Skinning the SpinnerList
sub-components
This section only explains how to access the SpinnerList
sub-components. Please read How to use the SpinnerList
component for full details about the skinning properties that are available on SpinnerList
components.
With a Theme
If you're creating a theme, you can target the DateTimeSpinner.DEFAULT_CHILD_STYLE_NAME_LIST
style name.
getStyleProviderForClass( SpinnerList )
.setFunctionForStyleName( DateTimeSpinner.DEFAULT_CHILD_STYLE_NAME_LIST, setDateTimeSpinnerListStyles );
The styling function might look like this:
private function setDateTimeSpinnerListStyles( list:SpinnerList ):void
{
var skin:Image = new Image( texture );
skin.scale9Grid = new Rectangle( 2, 3, 1, 6 );
list.backgroundSkin = skin;
}
You can override the default style name to use a different one in your theme, if you prefer:
spinner.customListStyleName = "custom-list";
You can set the function for the customListStyleName
like this:
getStyleProviderForClass( SpinnerList )
.setFunctionForStyleName( "custom-list", setDateTimeSpinnerCustomListStyles );
Without a Theme
If you are not using a theme, you can use listFactory
to provide skins for the list sub-components:
spinner.listFactory = function():SpinnerList
{
var list:SpinnerList = new SpinnerList();
//skin the lists here, if you're not using a theme
var skin:Image = new Image( texture );
skin.scale9Grid = new Rectangle( 2, 3, 1, 6 );
list.backgroundSkin = skin;
return list;
}